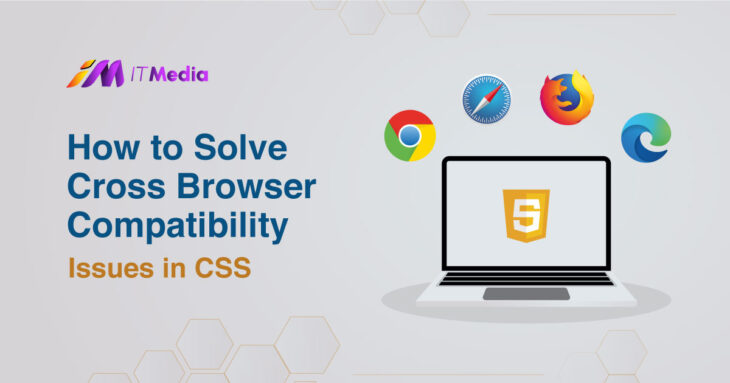
Cross-browser compatibility is important for web development because it ensures that users can access and interact with any website regardless of the browser they use.
One of the most difficult challenges in achieving it is dealing with the differences in how different browsers interpret and render CSS.
In this article, we will discuss the causes of cross-browser compatibility issues and how to resolve cross-browser compatibility issues in CSS.
Causes of Cross-Browser Compatibility Issues in CSS
Before we get into solutions, let’s first understand the root causes of cross-browser compatibility issues in CSS:
Differences in CSS Interpretation and Implementation Across Browsers
Each browser has its own rendering engine, which interprets and executes CSS differently.
For example, WebKit powers Safari, Chrome, and Opera, while Mozilla Firefox uses Gecko.
These differences can cause inconsistencies in how CSS rules are applied, resulting in visible inconsistencies between browsers.
Disparities in Browser Rendering Engines
Although most modern browsers have adopted the open-source WebKit rendering engine, there are some significant differences in how each browser renders web pages.
For example, Internet Explorer and Edge have their proprietary rendering engines, which can cause compatibility issues.
Outdated or Non-Standard CSS Features
Using outdated or non-standard CSS properties can cause compatibility issues.
Older browsers may not recognize the new CSS properties, leading to unpredictable styling or functionality.
Poorly Written CSS Code
Poorly structured or poorly written CSS code can contribute to cross-browser compatibility issues.
Errors in CSS code, such as typos or missing semicolons, can prevent styles from being applied correctly, resulting in inconsistent styling across browsers.
Solutions for Common Cross-Browser Compatibility Issues in CSS
We’ve discussed the main causes of cross-browser compatibility issues in CSS.
Let’s dive into practical solutions on how to solve cross-browser compatibility issues in CSS:
Using CSS Standards and Best Practices
Following CSS standards and best practices helps minimize compatibility issues.
Here are some tips to follow:
Following CSS Specifications and Recommendations From W3C
The World Wide Web Consortium (W3C) provides guidelines and specifications for CSS.
Make sure you follow these guidelines when writing CSS code.
Using Vendor-Prefixed Properties and Values
To ensure compatibility with different browsers, use vendor-prefixed properties and values.
For example, use `-webkit-` for WebKit browsers, `-moz-` for Mozilla Firefox, and `-ms-` for Microsoft browsers.
Vendor prefixes allow you to apply styles specifically for specific browsers, reducing compatibility issues.
Avoiding Outdated or Non-Standard CSS Features
Avoid using outdated or non-standard CSS properties. Instead, focus on using modern CSS features that enjoy broad support across most browsers.
If you want to use a non-standard feature, consider adding a vendor prefix or using a polyfill to ensure backward compatibility.
Testing and Debugging Techniques
Testing and debugging are important steps in identifying and resolving cross-browser compatibility issues.
Here are some tips to help you test and debug your CSS effectively:
Use Browser Developer Tools
All modern browsers come equipped with developer tools that allow you to inspect HTML and CSS elements, modify styles, and diagnose problems.
Familiarize yourself with these tools to quickly identify and resolve compatibility issues
Testing on Multiple Devices and Browsers
Test your website on different devices and browsers to identify potential compatibility issues.
You can use services like BrowserStack or Responsinator to test your website on different devices and browsers without needing physical access to them.
Debugging CSS with Chrome DevTools
Chrome DevTools provides excellent capabilities for debugging CSS.
You can inspect individual elements, adjust styles live, and analyze formatting issues effortlessly.
Fallback Options and Polyfills
Providing fallback styles for older browsers and using polyfills for missing CSS properties are two techniques that can help ensure cross-browser compatibility.
Providing Fallback Styles
When a browser does not support a particular CSS property, it is useful to provide a fallback option.
This ensures that the content still looks good, even if it doesn’t look exactly as intended.
Here’s an example of providing a fallback for a flex container:
“`css
.container {
display: flex;
flex-wrap: wrap;
}
.item {
flex: 1;
}
/* Fallback for older browsers */
.container {
display: block;
}
.item {
float: left;
width: 50%;
}
“`
In this example, we set the `.container` element to display as a flex container and the `.item` element to fill the container evenly.
However, for older browsers that don’t support flexbox, we set the `.container` element and `.item` element to float left and 50% width to display as block elements.
Using Polyfills
Polyfills are JavaScript files that replicate the behavior of a particular CSS property in browsers that don’t support it.
This allows you to use new CSS features without worrying about compatibility issues.
To use a polyfill, simply include the JavaScript file in your HTML or CSS file.
Graceful Degradation
Cross-browser compatibility is an important part of modern design. This involves creating flexible and adaptable layouts that can fit a variety of screen sizes and devices.
CSS media queries allow you to define different styles for different screen sizes and orientations, so your design looks great on all devices.
Here’s an example of a media query that targets screens with a maximum width of 768 pixels:
@media (max-width: 768px) {
/* Styles for screens with a max width of 768px */
body {
padding: 20px;
margin: 20px;
background-color: #f2f2f2;
}
/* Adjust the layout for smaller screens */
header, footer {
height: auto;
}
main {
flex-direction: column;
}
}
In this example, the media query targets screens with a maximum width of 768 pixels and applies alternative styles to the body, header, footer, and main elements. Layout adjustments ensure that content is displayed appropriately on small screens.
Best Practices for Writing Cross-Browser Compatible CSS
Here are some best practices to write cross-browser compatible CSS code:
A. Organizing CSS Code for Easier Maintenance and Updates
Keeping your CSS code organized is important for easy maintenance and updates.
Here are some tips for organizing your CSS code:
- Use a logical folder structure
- Use a consistent naming convention
- Break up large files
- Use a version control system
B. Commenting and Documenting CSS Code
Commenting and documenting your CSS code is necessary for communication with other developers and designers.
Here are some tips for commenting and documenting your CSS code:
- Use clear and concise comments to explain what each part of your code does.
- Document your code by including information about the purpose of each class, ID, and selector.
- Consider using a documentation tool like Dox or JSDoc to generate documentation for your code.
C. Using Sass or Less Preprocessors for More Efficient Coding
Sass and Less are popular preprocessors that can help you write more efficient CSS code.
Here are some benefits of using preprocessors:
- Preprocessors allow you to define variables for colors, fonts, and other styles, making it easier to maintain consistency throughout your code.
- Preprocessors allow you to nest selectors, making your code more readable and easier to maintain.
- Preprocessors allow you to define functions that can be reused throughout your code, reducing repetition and improving efficiency.
Tips for Writing Cleaner and More Maintainable CSS
Here are some additional tips for writing cleaner and more maintainable CSS:
- Use descriptive class names that accurately reflect the purpose of each element.
- Avoid using !important, as it can make it harder to override styles in other parts of your code. Instead, use a more specific selector to target the element you want to style.
- CSS frameworks like Bootstrap or Foundation provide pre-built CSS classes that you can use to speed up your development process.
Tools and Resources for Ensuring Cross-Browser Compatibility
In addition to best practices, there are many tools and resources available that can help you ensure cross-browser compatibility.
Here are some of our favorites:
- BrowserStack: It is a cloud-based platform that allows you to test your website on real devices and browsers.
- Can I Use…: It is a handy tool that allows you to check browser support for CSS features.
Conclusion
Cross-browser compatibility in CSS requires a multifaceted approach.
By understanding the challenges, implementing best practices, and being proactive in maintenance, developers can create websites that provide a consistent and enjoyable experience across all browsers.
# FAQs
Q1: How can I quickly identify cross-browser compatibility issues during development?
A1: Utilize browser developer tools and online testing platforms to detect issues early in the development process.
Q2: Are there specific CSS properties more prone to compatibility problems?
A2: Yes, certain properties, such as those involving layout and positioning, are more likely to cause compatibility issues.
Q3: Should I use browser-specific hacks to address compatibility problems?
A3: Use browser-specific hacks cautiously and only when absolutely necessary, considering the potential downsides.
Q4: How often should I update my CSS code for cross-browser compatibility?
A4: Regular updates are essential, especially after browser updates or changes in CSS specifications.
Q5: What role does user feedback play in solving compatibility issues?
A5: User feedback is valuable for identifying unforeseen compatibility problems, providing insights for continuous improvement.